Notice
Recent Posts
Recent Comments
Link
맥에서 오픈소스로
아두이노 <-> PC 간 UDP 트리거 신호 전송 성공! 본문
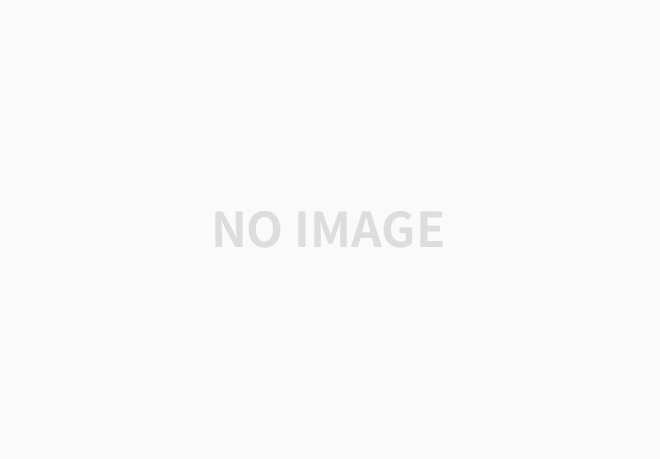
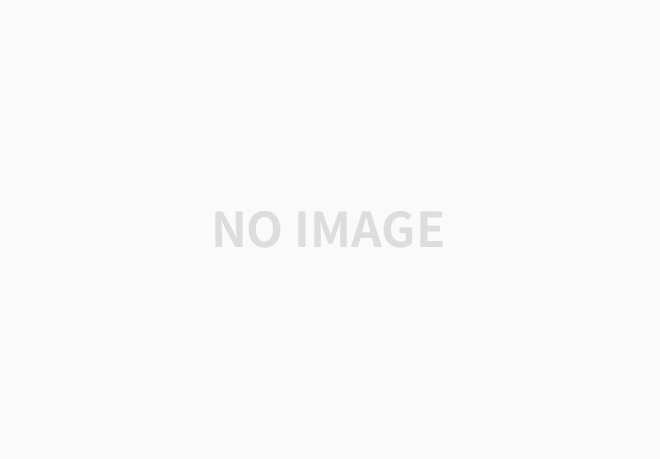
#include <SPI.h>
#include <Ethernet.h>
#include <EthernetUdp.h>
// MAC 주소 설정 (임의의 주소)
byte mac[] = { 0xDE, 0xAD, 0xBE, 0xEF, 0xFE, 0xED };
// 아두이노의 IP 주소 설정
IPAddress ip(192, 168, 0, 177);
unsigned int localPort = 8888; // 로컬 포트
// UDP 통신을 위한 변수
EthernetUDP Udp;
const int buttonPin = 2; // 버튼 핀 번호
int lastButtonState = HIGH;
void setup() {
Serial.begin(9600);
pinMode(buttonPin, INPUT_PULLUP);
// 이더넷 초기화
Ethernet.begin(mac, ip);
Udp.begin(localPort);
// 초기화 대기
delay(1500);
}
void loop() {
int buttonState = digitalRead(buttonPin);
if (buttonState != lastButtonState) {
if (buttonState == LOW) {
// 버튼이 눌렸을 때 "1" 전송
Udp.beginPacket(IPAddress(192, 168, 0, 51), 8888); // 목적지 IP와 포트
Udp.write("1");
Udp.endPacket();
}
delay(50); // 디바운싱
}
lastButtonState = buttonState;
}
- Arduino Code
import sys
import socket
from PyQt5.QtWidgets import QApplication, QMainWindow, QWidget, QMessageBox
from PyQt5.QtCore import QTimer
from PyQt5.QtGui import QPalette, QColor
class MainWindow(QMainWindow):
def __init__(self):
super().__init__()
self.initUI()
self.initUDP()
def initUI(self):
self.setGeometry(100, 100, 400, 300)
self.setWindowTitle('Arduino UDP Color Change Demo')
# 중앙 위젯 설정
central_widget = QWidget()
self.setCentralWidget(central_widget)
# 초기 색상 설정
self.current_color = 0 # 0: 흰색, 1: 빨간색
self.updateColor()
def initUDP(self):
try:
# UDP 소켓 생성
self.sock = socket.socket(socket.AF_INET, socket.SOCK_DGRAM)
self.sock.bind(('192.168.0.51', 8888)) # 컴퓨터의 IP와 포트
self.sock.setblocking(False)
print("UDP 소켓 생성 성공!")
# 타이머 설정
self.timer = QTimer()
self.timer.timeout.connect(self.readUDP)
self.timer.start(100) # 100ms 마다 체크
except Exception as e:
QMessageBox.critical(self, "오류", f"UDP 소켓 생성 실패: {str(e)}")
self.close()
def readUDP(self):
try:
data, addr = self.sock.recvfrom(1024)
if data:
received = data.decode().strip()
print(f"수신된 데이터: {received}") # 디버깅용
if received == "1":
self.current_color = 1 - self.current_color # 색상 토글
self.updateColor()
except BlockingIOError:
pass # 데이터가 없을 때는 무시
except Exception as e:
print(f"데이터 읽기 오류: {str(e)}")
def updateColor(self):
palette = self.centralWidget().palette()
if self.current_color == 0:
palette.setColor(QPalette.Window, QColor('white'))
print("색상 변경: 흰색")
else:
palette.setColor(QPalette.Window, QColor('red'))
print("색상 변경: 빨간색")
self.centralWidget().setAutoFillBackground(True)
self.centralWidget().setPalette(palette)
def closeEvent(self, event):
try:
if hasattr(self, 'sock'):
self.sock.close()
print("UDP 소켓 종료")
except Exception as e:
print(f"소켓 종료 오류: {str(e)}")
event.accept()
if __name__ == '__main__':
app = QApplication(sys.argv)
window = MainWindow()
window.show()
sys.exit(app.exec_())
'기타' 카테고리의 다른 글
파이썬 자동 들여쓰기 패키지 (0) | 2025.02.05 |
---|---|
ArduinoPLC <-> PC 간 UDP 통신 OK (0) | 2025.01.09 |
아두이노 <-> PC 간 시리얼 통신 (0) | 2025.01.07 |
끌로드(chatGPT) 시대에 파이썬 언어의 들여쓰기 유감 (1) | 2024.10.22 |
아침 냥이 (1) | 2024.10.18 |